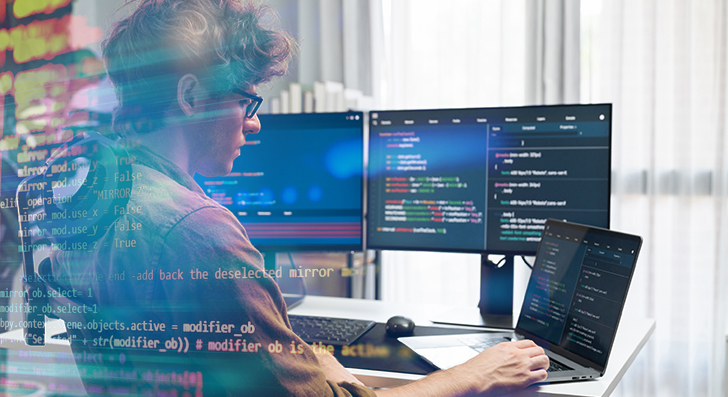
Scalability usually means your application can manage growth—extra people, extra knowledge, and a lot more site visitors—with out breaking. To be a developer, making with scalability in mind will save time and anxiety later. Below’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability isn't really some thing you bolt on afterwards—it should be aspect of one's system from the beginning. Quite a few applications fall short once they improve quick mainly because the original style and design can’t deal with the additional load. To be a developer, you should Imagine early about how your procedure will behave under pressure.
Start out by designing your architecture to get adaptable. Steer clear of monolithic codebases wherever every thing is tightly linked. In its place, use modular style or microservices. These designs crack your application into smaller, impartial sections. Each module or support can scale By itself without the need of affecting The entire technique.
Also, give thought to your database from day a single. Will it will need to take care of one million customers or simply a hundred? Select the appropriate style—relational or NoSQL—based on how your information will expand. System for sharding, indexing, and backups early, Even when you don’t need them nonetheless.
Another essential level is in order to avoid hardcoding assumptions. Don’t publish code that only will work less than present-day conditions. Consider what would transpire If the person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use style patterns that assistance scaling, like message queues or function-driven techniques. These aid your app deal with much more requests with out getting overloaded.
When you build with scalability in your mind, you are not just making ready for achievement—you are lowering long term headaches. A perfectly-prepared process is simpler to keep up, adapt, and expand. It’s much better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the ideal databases is actually a important Portion of building scalable purposes. Not all databases are created the identical, and using the Erroneous one can gradual you down as well as trigger failures as your app grows.
Start by knowledge your info. Can it be hugely structured, like rows inside a desk? If Indeed, a relational database like PostgreSQL or MySQL is a great suit. These are generally powerful with interactions, transactions, and consistency. In addition they help scaling techniques like examine replicas, indexing, and partitioning to handle additional targeted traffic and data.
In the event your knowledge is a lot more versatile—like person activity logs, product or service catalogs, or documents—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at managing substantial volumes of unstructured or semi-structured information and might scale horizontally extra very easily.
Also, take into consideration your study and produce styles. Have you been accomplishing plenty of reads with less writes? Use caching and read replicas. Have you been managing a heavy create load? Check into databases which can deal with substantial generate throughput, or even occasion-centered information storage techniques like Apache Kafka (for momentary data streams).
It’s also intelligent to Consider in advance. You might not require Innovative scaling options now, but deciding on a database that supports them signifies you received’t have to have to modify afterwards.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your knowledge determined by your obtain patterns. And usually keep track of database efficiency as you expand.
In a nutshell, the best databases is dependent upon your app’s construction, speed demands, And just how you assume it to improve. Acquire time to choose correctly—it’ll preserve plenty of problems later.
Optimize Code and Queries
Fast code is key to scalability. As your app grows, every small hold off provides up. Badly created code or unoptimized queries can slow down overall performance and overload your method. That’s why it’s important to Establish efficient logic from the beginning.
Start out by composing thoroughly clean, easy code. Avoid repeating logic and take away everything needless. Don’t choose the most advanced Resolution if a simple a single works. Keep the features brief, concentrated, and simple to test. Use profiling instruments to seek out bottlenecks—locations where by your code normally takes also long to operate or makes use of too much memory.
Following, take a look at your databases queries. These frequently gradual issues down much more than the code by itself. Be certain Each individual query only asks for the info you actually need to have. Steer clear of Pick out *, which fetches every thing, and as a substitute choose unique fields. Use indexes to speed up lookups. And stay clear of carrying out a lot of joins, In particular across massive tables.
For those who recognize a similar information currently being asked for repeatedly, use caching. Keep the effects briefly applying instruments like Redis or Memcached so that you don’t should repeat expensive operations.
Also, batch your database functions after you can. Rather than updating a row one by one, update them in teams. This cuts down on overhead and would make your application more effective.
Remember to test with huge datasets. Code and queries that operate great with a hundred records may crash whenever they have to manage one million.
To put it briefly, scalable applications are fast apps. Maintain your code restricted, your queries lean, and use caching when wanted. These techniques assistance your software continue to be sleek and responsive, at the same time as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more consumers plus much more targeted visitors. If everything goes through one server, it'll rapidly become a bottleneck. That’s where load balancing and caching come in. Both of these instruments enable maintain your app quick, steady, and scalable.
Load balancing spreads incoming targeted traffic across numerous servers. Rather than one server doing many of the do the job, the load balancer routes people to diverse servers depending on availability. This implies no single server receives overloaded. If one particular server goes down, the load balancer can send out traffic to the Other people. Tools like Nginx, HAProxy, or cloud-dependent remedies from AWS and Google Cloud make this simple to set check here up.
Caching is about storing facts briefly so it can be reused promptly. When end users request a similar data once more—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You may serve it within the cache.
There are 2 typical different types of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for speedy accessibility.
two. Consumer-facet caching (like browser caching or CDN caching) retailers static data files near to the person.
Caching minimizes databases load, improves pace, and makes your app extra productive.
Use caching for things that don’t adjust often. And constantly make sure your cache is up to date when details does modify.
Briefly, load balancing and caching are easy but strong tools. Collectively, they assist your application deal with additional users, continue to be fast, and Recuperate from challenges. If you propose to develop, you require both.
Use Cloud and Container Resources
To develop scalable purposes, you need resources that allow your application mature easily. That’s in which cloud platforms and containers can be found in. They give you versatility, minimize set up time, and make scaling Substantially smoother.
Cloud platforms like Amazon Web Solutions (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and companies as you would like them. You don’t have to purchase hardware or guess potential capability. When targeted traffic boosts, you are able to include much more sources with only a few clicks or instantly making use of automobile-scaling. When site visitors drops, it is possible to scale down to save cash.
These platforms also supply providers like managed databases, storage, load balancing, and safety resources. You'll be able to concentrate on developing your app as opposed to handling infrastructure.
Containers are An additional key tool. A container offers your application and every little thing it has to run—code, libraries, configurations—into just one unit. This makes it easy to maneuver your app in between environments, from a notebook on the cloud, devoid of surprises. Docker is the most well-liked tool for this.
Once your application utilizes multiple containers, instruments like Kubernetes allow you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular component within your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into solutions. You could update or scale areas independently, that is perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container instruments indicates you may scale quickly, deploy easily, and Get well quickly when troubles happen. In order for you your app to expand without boundaries, begin employing these tools early. They preserve time, cut down threat, and make it easier to stay focused on making, not fixing.
Check All the things
In the event you don’t keep an eye on your software, you won’t know when issues go Mistaken. Checking helps you see how your app is performing, spot troubles early, and make improved decisions as your app grows. It’s a crucial Component of setting up scalable methods.
Commence by monitoring primary metrics like CPU use, memory, disk House, and response time. These tell you how your servers and providers are undertaking. Instruments like Prometheus, Grafana, Datadog, or New Relic will let you collect and visualize this information.
Don’t just check your servers—observe your application too. Keep an eye on how long it will take for customers to load webpages, how often mistakes take place, and in which they arise. Logging equipment like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring inside your code.
Put in place alerts for critical troubles. By way of example, When your response time goes previously mentioned a limit or perhaps a assistance goes down, it is best to get notified promptly. This will help you correct concerns quick, frequently before users even see.
Checking is additionally helpful whenever you make modifications. If you deploy a completely new attribute and see a spike in errors or slowdowns, you could roll it back again prior to it causes authentic hurt.
As your app grows, visitors and details raise. Without having checking, you’ll miss out on signs of hassle right up until it’s as well late. But with the ideal equipment in place, you keep in control.
Briefly, monitoring can help you keep your application dependable and scalable. It’s not pretty much spotting failures—it’s about being familiar with your technique and making sure it works perfectly, even under pressure.
Remaining Feelings
Scalability isn’t just for massive companies. Even modest apps need to have a solid foundation. By coming up with cautiously, optimizing correctly, and using the proper applications, you'll be able to Establish apps that increase smoothly without having breaking stressed. Start tiny, Assume large, and Create good.